The event loop is a fundamental concept in JavaScript, especially crucial for understanding how asynchronous operations are handled. JavaScript is single-threaded, meaning it executes one operation at a time. The event loop allows JavaScript to perform non-blocking operations, despite being single-threaded, by offloading operations to the browser or the Node.js runtime. Imagine it as a queue system: events like user interactions or network requests are added to the queue, and the engine processes them one by one. This allows JavaScript to handle non-blocking tasks without freezing, keeping the application responsive even while waiting for data or other operations.
How do Event loop work?
1. Call Stack:
- The call stack is a data structure that records where in the program we are.
- When a function is called, it’s added to the top of the stack.
- When a function returns, it’s removed from the top of the stack.
2. Web APIs (or Node APIs):
- For browser environments, this includes things like setTimeout, DOM events, HTTP requests, etc.
- For Node.js, this includes file system operations, network requests, etc.
- These APIs handle asynchronous operations and provide a way to offload tasks from the call stack.
3. Callback Queue (or Task Queue):
- When an asynchronous operation completes, its callback function is moved to the callback queue.
- This queue holds the callbacks ready to be executed.
4. Event Loop:
- The event loop is a constantly running process that checks if the call stack is empty.
- If the call stack is empty and there are callbacks in the callback queue, the event loop dequeues the first callback and pushes it onto the call stack, where it is executed.
Steps of the Event Loop:
- Check Call Stack: If the call stack is not empty, the event loop does nothing and waits until it becomes empty.
- Check Callback Queue: If the call stack is empty, the event loop checks the callback queue. If there are pending callbacks, it dequeues the first one and pushes it onto the call stack.
- Execute Callback: The callback is executed by the call stack.
- Repeat: The event loop continues this process, ensuring that the JavaScript code runs smoothly without blocking.
console.log('Start');
setTimeout(() => {
console.log('Callback from setTimeout');
}, 2000);
console.log('End');
Execution Flow:
console.log('Start')
is executed, outputting “Start”.setTimeout
is called, which sets a timer for 2000 milliseconds. The callback is offloaded to the Web APIs.console.log('End')
is executed, outputting “End”.- After 2000 milliseconds, the
setTimeout
callback is moved to the callback queue. - The event loop checks the call stack, finds it empty, and pushes the callback onto the call stack.
- The callback from
setTimeout
is executed, outputting “Callback from setTimeout”.
This sequence allows JavaScript to handle asynchronous operations without blocking the main thread, making it suitable for tasks like network requests, event handling, and more.
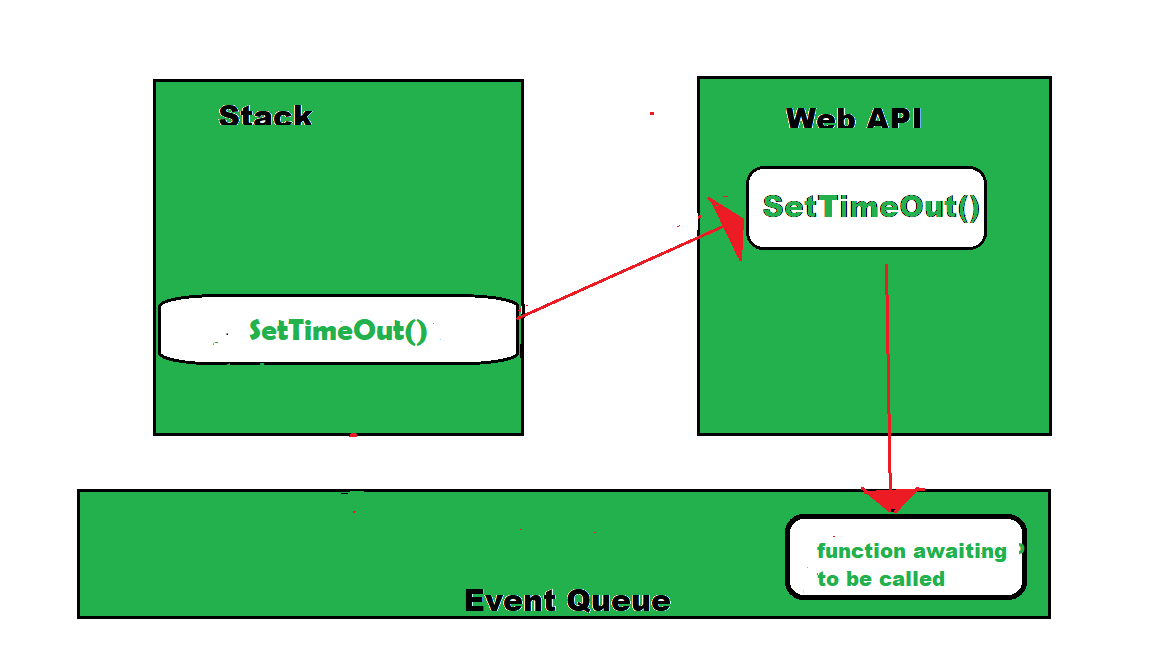
Disclaimer: Some content on this site is derived from or inspired by resources available on other websites. We gratefully acknowledge these sources.
References:
– https://www.nkgtech.com/video/event-loop-concept/
– https://www.geeksforgeeks.org/what-is-an-event-loop-in-javascript/
– Event Loop, Call stack, Callback queue explained by Philip Roberts in JSConf EU